Week2
r = sqrt(100.0); k = pow(x,y); i = strlen(str1); v = strcmp(str1,str2); n = atoi(str1);
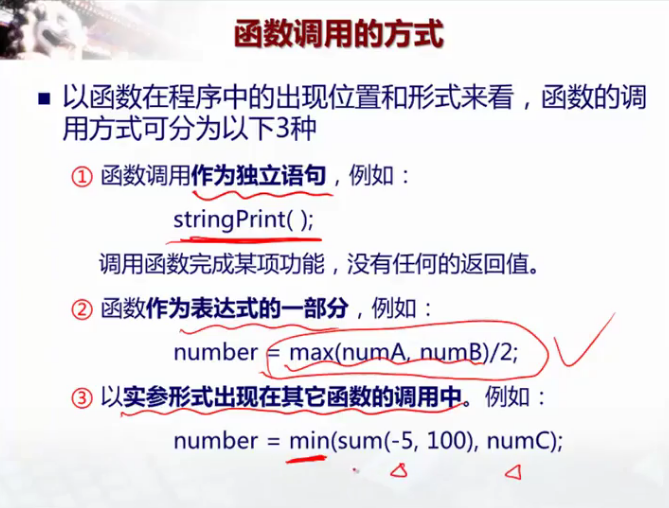
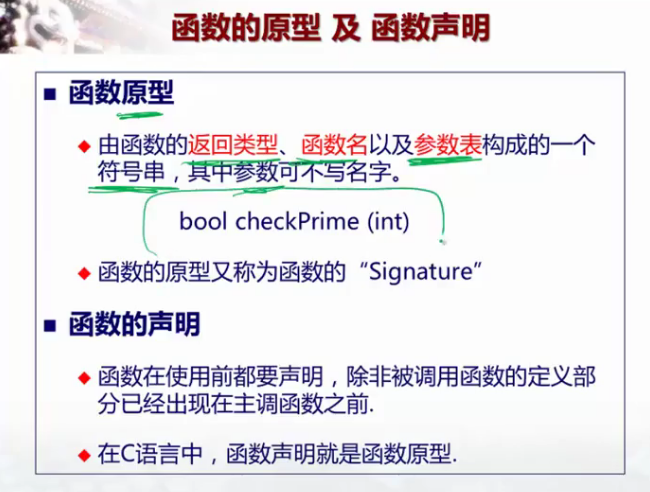
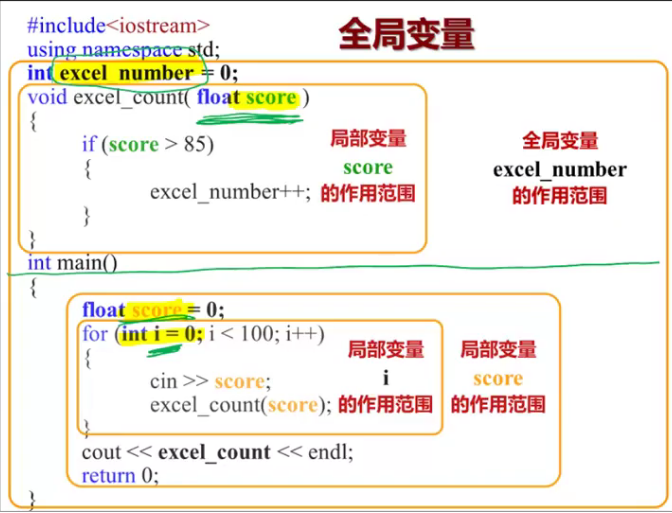
数组的名字 = 数组的地址 int a[2]; cout << a <<endl; //a is the address of a array
Week4 Pointer note:
取地址运算符:“&”
int c = 76; cout<<&c<<endl; cout<<sizeof(&c)<<endl; //4 bytes
— — — — —
int c = 76; int *pointer; //定义名字为pointer的指针变量 pointer = &c; //将变量c的地址赋值给指针变量pointer //赋值后,称指针变量pointer指向了变量c
— — — — — — — — — — — — —
int c = 76; int *pointer = &c; *pointer:所指向的存储单元的内容:变量c
— — — — — — — — — — — —
#include<iostream> using namespace std; int main() { int iCount = 18; int *iPtr = &iCount; *iPtr = 58; cout << iCount << endl; cout << iPtr << endl; cout << &iCount << endl; cout << *iPtr << endl; cout << &iPtr << endl; return 0; }
— — — — — —
#include<iostream> using namespace std; int main() { int a = 0, b = 0, temp; int *p1 = NULL, *p2 = NULL; cin >> a >> b; p1 = &a; p2 = &b; if (*p1 < *p2) { temp = *p1; *p1 = *p2; *p2 = temp; } cout << "max= " << *p1 << ",min= " << *p2 << endl; return 0; }
— — — — — — —
运算符优先级:后置++ -- 高于 (前置++-- 逻辑非 (!)* & ) 从右到左
#include<iostream> using namespace std; int main() { int a[5] = { 1,2,3,4,5 }; int *p = &a[3]; cout << *p << endl; *p = 100; cout << a[3] << endl; return 0; }
— — — — — — — — — — —
#include<iostream> using namespace std; int main() { int a[5] = { 1,2,3,4,5 }; cout << a << endl; cout << *a << endl; cout << &a[0] << endl; cout << a[0] << endl; return 0; }
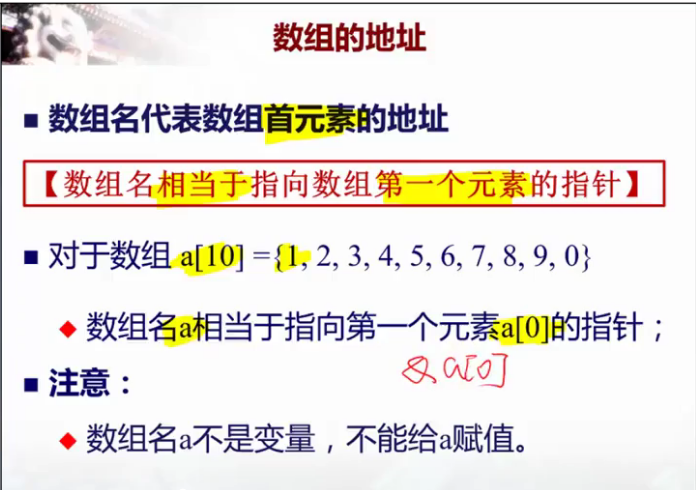
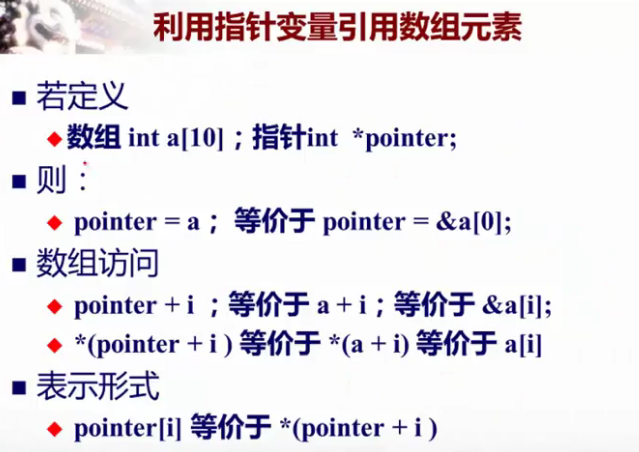
#include<iostream> #include<iomanip> using namespace std; int main() { int a[10], *p = NULL, *q = NULL, temp; for (p = a; p < a + 10; p++) cin >> *p; for (p = a, q = a + 9; p < q; p++, q--) { temp = *p; *p = *q; *q = temp; } for (p = a; p < a + 10; p++) cout << setw(3) << *p; return 0; }
— — — — — — — — —
#include<iostream>
using namespace std; int main() { int a[3][4] = { 1,2,3,4,5,6,7,8,9,10,11,12 }; int(*p)[4], i, j; p = a; cin >> i >> j; cout << *(*(p + i) + j) << endl; .
return 0; }
Week5:
char c[6]={'h','e','l','l','o','\0'} char *pc = c; cout<<c<<endl; //hello cout<<pc<<endl; //hello
cout<<static_cast<void*>(c)<<endl; //address cout<<static_cast<void*>(pc)<<endl; //address
— — — — — — — — —
#include<iostream> using namespace std; int main() { char buffer[10] = "ABC"; char *pc; pc = "hello"; cout << pc << endl; pc++; cout << pc << endl; cout << *pc << endl; pc = buffer; cout << pc; return 0; } output: hello ello e ABC
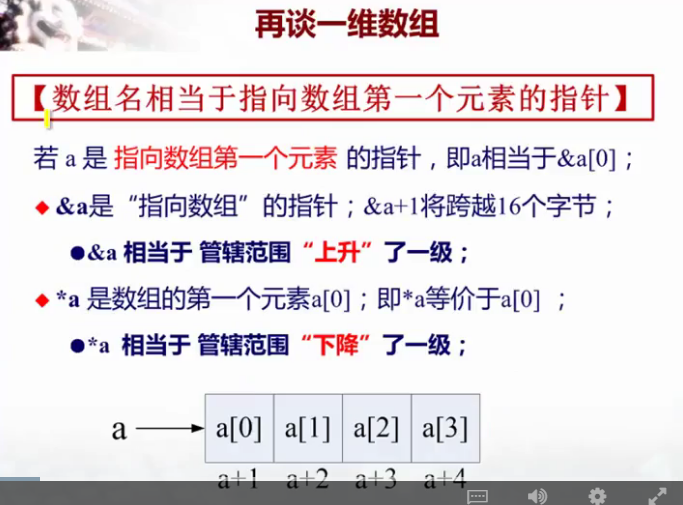
Week 7:
Struct: 结构体数据类型的特性与普通数据类型的特性是一致的。
#include<iostream> using namespace std;
struct student { int id_num; char name[10]; };
void renew(student *one) { one->id_num = 20130000 + one->id_num; //one->id_num is equivalent to (*one).id_num for (int i = 0; one->name[i] != '\0'; i++) one->name[i] = toupper(one->name[i]); }
int main() { student mike = { 123,{'m','i','k','e','\0'} }; renew(&mike); cout << mike.id_num << " " << mike.name; return 0; }
— — — — — — — — — —
#include<iostream> using namespace std;
struct student { int id_num; char name[10]; };
int main() { student myclass[3] = { 123,{'m','i','k','e','\0'}, 133,{'t','o','m','\0'}, 143,{'j','a','c','k','\0'} }; student *one = myclass; cout << (*one).id_num << " " << (*one).name << endl; one++; cout << one->id_num << " " << one->name << endl; return 0; }
— — — — — — — — — — — — —
LinkedList:
Creation:
#include<iostream> using namespace std;
struct student { int id; student *next; };
student *create() { student *head, *temp; int num, n = 0; head = new student; temp = head; cin >> num; while (num != -1) { n++; temp->id = num; temp->next = new student; temp = temp->next; cin >> num; } if (n == 0)head = NULL; else temp->next = NULL; return head; }
int main() { return 0; }
— — — — — — — — — — — — — — —
Delete
student *dele(student *head, int n) { student *temp, *follow; temp = head; if (head == NULL) { return head; } if (head-> num == n) { head = head->next; delete temp; return(head); } while (temp != NULL&&temp->num != n) { follow = temp; temp = temp->next; } if (temp == NULL) cout << "not found"; else { follow->next = temp->next; delete temp; } return head;
— — — — — — — — — —
Insert:
#include<iostream> using namespace std;
struct student { int id,num; student *next; };
student *create() { student *head, *temp; int num, n = 0; head = new student; temp = head; cin >> num; while (num != -1) { n++; temp->id = num; temp->next = new student; temp = temp->next; cin >> num; } if (n == 0)head = NULL; else temp->next = NULL; return head; }
student *dele(student *head, int n) { student *temp, *follow; temp = head; if (head == NULL) { return head; } if (head-> num == n) { head = head->next; delete temp; return(head); } while (temp != NULL&&temp->num != n) { follow = temp; temp = temp->next; } if (temp == NULL) cout << "not found"; else { follow->next = temp->next; delete temp; } return head; }
student *insert(student *head, int n) { student *temp, *unit, *follow; temp = head; unit = new student; unit->num = n; unit->next = NULL; if (head == NULL) { head = unit; return(head); } while ((temp->next != NULL) && (temp->num < n)) { follow = temp; temp = temp->next; } if (temp == head) { unit->next = head; head = unit; } else { if (temp->next == NULL)temp->next = unit; else { follow->next = unit; unit->next=temp; } } return(head); }
int main() { student *pointer = create(); while (pointer->next!=NULL) { cout << pointer->id << endl; pointer = pointer->next; } return 0; }
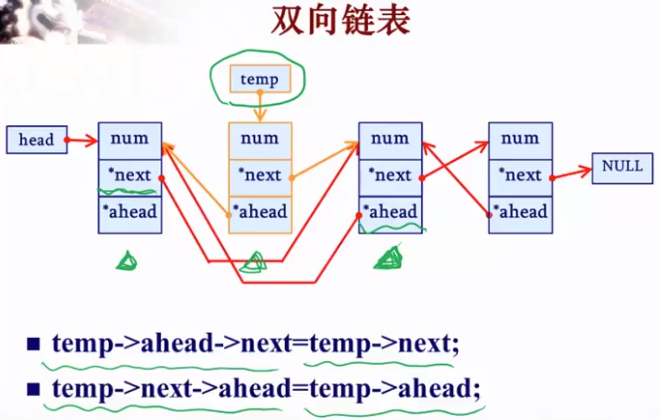
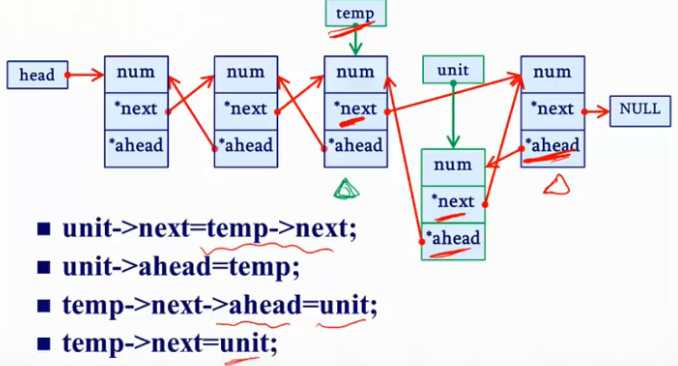
#include <iostream> #include <cstring> using namespace std;
int m, n;//n行m列 char map[101][101];//地图矩阵 int already[101][101];//已走地图记忆 int min_count = 0;
void cross(int u, int v, int i, int j) { int t = already[u][v]; if (u == i && v == j)//起点即为到达终点 { min_count = t; } t++;
if (v < m - 1 && map[u][v + 1] != '#' && already[u][v + 1]>t)//在迷宫内、右侧非墙且此点没有走过 { already[u][v + 1] = t;//标记此点为第t步 cross(u, v + 1, i, j);//以此点为起点继续走 } if (u > 0 && map[u - 1][v] != '#' && already[u - 1][v]>t) { already[u - 1][v] = t; cross(u - 1, v, i, j); } if (v > 0 && map[u][v - 1] != '#' && already[u][v - 1]>t) { already[u][v - 1] = t; cross(u, v - 1, i, j); } if (u < n - 1 && map[u + 1][v] != '#' && already[u + 1][v]>t) { already[u + 1][v] = t; cross(u + 1, v, i, j); } }
int main() { int startx, starty, endx, endy; cin >> n >> m; for (int i = 0; i<n; i++) { for (int j = 0; j<m; j++) { cin >> map[i][j]; if (map[i][j] == 'S') { startx = i; starty = j; } if (map[i][j] == 'T') { endx = i; endy = j; } } } memset(already, 1, sizeof(already)); already[startx][starty] = 0; cross(startx, starty, endx, endy); cout << min_count << endl; return 0; }
C++ 引用 vs 指针
引用很容易与指针混淆,它们之间有三个主要的不同:
引用很容易与指针混淆,它们之间有三个主要的不同:
不存在空引用。引用必须连接到一块合法的内存。
一旦引用被初始化为一个对象,就不能被指向到另一个对象。指针可以在任何时候指向到另一个对象。
引用必须在创建时被初始化。指针可以在任何时间被初始化。
一旦引用被初始化为一个对象,就不能被指向到另一个对象。指针可以在任何时候指向到另一个对象。
引用必须在创建时被初始化。指针可以在任何时间被初始化。
#include <iostream> using namespace std; int main () { // 声明简单的变量 int i; double d; // 声明引用变量 int& r = i; double& s = d; i = 5; cout << "Value of i : " << i << endl; cout << "Value of i reference : " << r << endl; d = 11.7; cout << "Value of d : " << d << endl; cout << "Value of d reference : " << s << endl; return 0; }
No comments:
Post a Comment